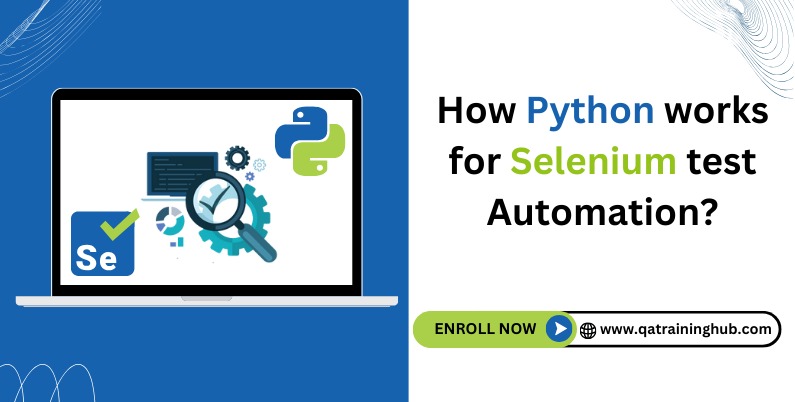
Python is a popular programming language for Selenium test automation due to its simplicity, readability, and a wide range of libraries and frameworks available. Selenium is a suite of tools for automating web browsers, and Python can be used to interact with Selenium WebDriver, which is the primary component for automating web interactions. Here’s how Python works for Selenium test automation:
1.Installing Selenium:
First, you need to install Selenium and a web driver for your preferred browser (e.g., Chrome, Firefox, Edge). You can use a package manager like pip to install Selenium:
Example
pip install selenium
2.Importing Selenium in Python:
Import the necessary Selenium modules into your Python script. You typically need to import the webdriver module and other related modules like Keys for keyboard actions:
Example
from selenium import webdriver from selenium.webdriver.common.keys import Keys
3.Initializing the WebDriver:
You need to create an instance of the WebDriver for the specific browser you want to automate. For Example, to use Chrome:
driver = webdriver.Chrome(executable_path=’path/to/chromedriver’)
Replace ‘path/to/chromedriver’ with the actual path to the Chrome WebDriver executable.
4.Navigating to Web Pages:
You can use the WebDriver to open web pages by providing the URL:
Example
driver.get(‘https://www.example.com’)
5.Locating Web Elements:
Selenium provides various methods to locate HTML elements on a web page, such as find_element_by_id, find_element_by_name, find_element_by_xpath, and more. For example:
search_box = driver.find_element_by_name(‘q’)
6.Interacting with Web Elements:
You can interact with web elements by sending text, clicking buttons, or performing other actions. For
Example:
search_box.send_keys(‘Selenium automation’) search_box.send_keys(Keys.RETURN)
7.Assertions and Validation:
You can use Python’s assertion mechanisms (e.g., assert statements) to validate that elements or conditions on the web page behave as expected. For instance, checking if a specific element exists:
Example:
assert “Selenium automation” in driver.page_source
8.Handling Pop-ups and Alerts:
Selenium allows you to handle various pop-ups, alerts, and dialogs that may appear during web interactions.
9.Closing and Quitting the WebDriver:
It’s essential to close and quit the WebDriver when you’re done with your automation tasks to release system resources:
driver.close() # Closes the current browser window
driver.quit() # Quits the WebDriver and closes all associated windows
10.Running Tests:
You can organize your Selenium test automation code into test cases using testing frameworks like unittest, pytest, or behave. These frameworks provide features for setting up, running, and reporting on tests.
11.Reporting and Logging:
To monitor and report on the execution of your test cases, you can use Python’s built-in logging or integrate with specialized reporting tools and frameworks.
12.Continuous Integration (CI):
Many organizations integrate Selenium test automation with CI/CD pipelines to automate testing as part of the software development process.
Python’s simplicity and the extensive capabilities of Selenium make it a powerful combination for automating web testing and interacting with web applications programmatically. It’s widely used in the software testing industry for web application test automation.
In conclusion, Python with Selenium is a formidable combination for automating web testing, and it’s highly regarded in the software testing industry for its simplicity, readability, and the wealth of libraries and frameworks available. QA Training Hub offers the best Selenium with Python course training, empowering aspiring testers and quality assurance professionals to harness the full potential of this powerful automation tool.